Numerical Partial Differential Equation II: Stability Analysis#
Numerical Methods for the Advection Equation#
In the previous section, we explored the Forward Time Centered Space (FTCS) scheme, a fundamental explicit finite difference method for solving the linear advection equation:
The FTCS scheme approximates the time derivative using a forward difference and the spatial derivatives using centered differences.
While straightforward to implement, the FTCS method is unconditionally unstable. We also provided some Python code to demonstrate the application of the FTCS scheme to a sinusoidal initial condition:
import numpy as np
import matplotlib.pyplot as plt
# Parameters
c = 1.0 # Advection speed
L = 1.0 # Domain length
T = 1.25 # Total time
nx = 100 # Number of spatial points
dx = L / nx # Spatial step size
dt = 0.001 # Initial time step size
nt = int(T / dt) # Number of time steps
# Stability parameter
sigma = c * dt / dx
print(f"Courant number (sigma): {sigma}")
# Spatial grid
x = np.linspace(0, L, nx)
u_initial = np.sin(2 * np.pi * x) # Initial condition: sinusoidal wave
# Initialize solution array
u = u_initial.copy()
# Time-stepping loop
for n in range(nt):
# Apply periodic boundary conditions using np.roll
u_new = u - (c * dt / (2 * dx)) * (np.roll(u, -1) - np.roll(u, 1))
u = u_new
# Analytical solution
u_exact = np.sin(2 * np.pi * (x - c * T))
# Plotting the results
plt.figure(figsize=(12, 6))
plt.plot(x, u_initial, label='Initial Condition', linestyle='--')
plt.plot(x, u_exact, label='Exact Solution', linewidth=2)
plt.plot(x, u, label='FTCS Scheme', linestyle=':', linewidth=2)
plt.xlabel('x')
plt.ylabel('u')
plt.legend()
plt.grid(True)
Courant number (sigma): 0.1
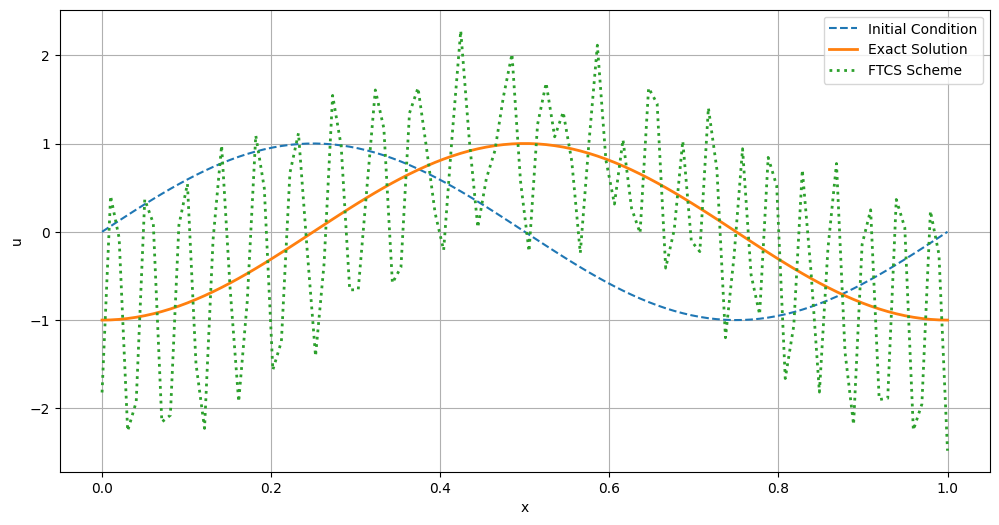
The simulation results illustrate how the FTCS scheme evolves the initial sinusoidal wave over time. However, due to its unconditional stability, numerical instability always occur.
To overcome the limitations of the FTCS scheme, we introduce two more robust finite difference methods: the Upwind Scheme and the Lax-Wendroff Scheme. These methods enhance stability and accuracy, making them more suitable for solving advection-dominated problems.
Upwind Scheme#
The Upwind Scheme is a finite difference method specifically designed to handle advection-dominated problems more effectively than symmetric schemes like FTCS. By incorporating the direction of wave propagation into the discretization of spatial derivatives, the upwind method enhances numerical stability and reduces non-physical oscillations.
In advection processes, information propagates in a specific direction determined by the flow velocity
The upwind scheme discretizes the spatial derivative based on the sign of the advection speed
Assuming
where:
is the numerical approximation of at spatial index and time level , and are the time and spatial step sizes, respectively.
The following Python code implements the upwind scheme to solve the linear advection equation for a sinusoidal initial condition.
import warnings
# Parameters
c = 1.0 # Advection speed
L = 1.0 # Domain length
T = 1.25 # Total time
nx = 100 # Number of spatial points
dx = L / nx # Spatial step size
dt = 0.001 # Initial time step size
nt = int(T / dt) # Number of time steps
# Stability parameter
sigma = c * dt / dx
print(f"Courant number (sigma): {sigma}")
# Check CFL condition
if sigma > 1:
warnings.warn(f"CFL condition violated: sigma = {sigma} > 1. Please reduce dt or increase dx.")
# Spatial grid
x = np.linspace(0, L, nx, endpoint=False)
u_initial = np.sin(2 * np.pi * x) # Initial condition: sinusoidal wave
# Initialize solution array
u = u_initial.copy()
# Time-stepping loop using Upwind scheme
for n in range(nt):
# Apply periodic boundary conditions using np.roll
u_new = u - sigma * (u - np.roll(u, 1))
u = u_new
# Analytical solution
u_exact = np.sin(2 * np.pi * (x - c * T))
# Plotting the results
plt.figure(figsize=(12, 6))
plt.plot(x, u_initial, label='Initial Condition', linestyle='--')
plt.plot(x, u_exact, label='Exact Solution', linewidth=2)
plt.plot(x, u, label='Upwind Scheme', linestyle=':', linewidth=2)
plt.xlabel('x')
plt.ylabel('u')
plt.legend()
plt.grid(True)
Courant number (sigma): 0.1
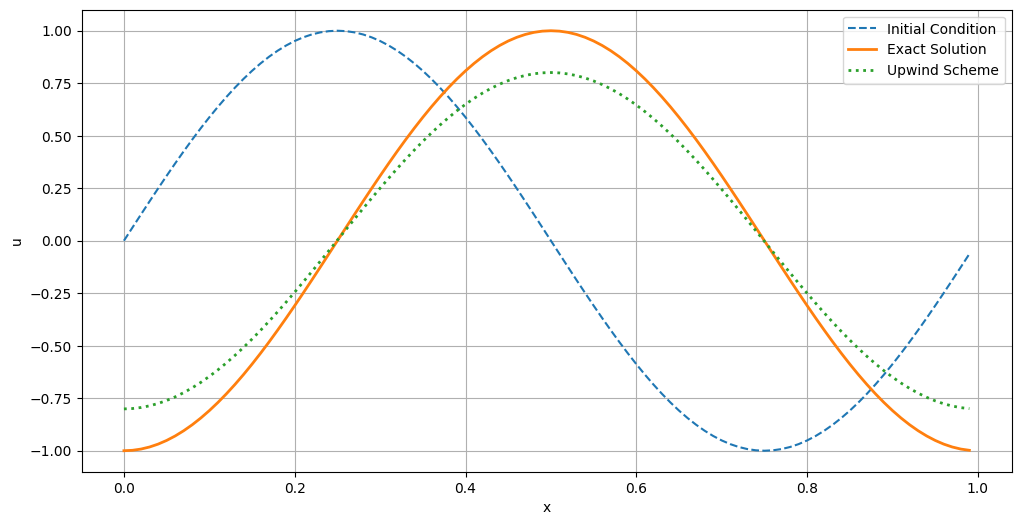
Von Neumann Stability Analysis#
Von Neumann Stability Analysis assesses the stability of numerical schemes by examining how Fourier modes are amplified or damped during the simulation.
Assume the numerical solution can be expressed as:
where:
is the amplification factor, is the wave number, .
Substitute
Divide both sides by
Using Euler’s formula and separate the real and imaginary parts:
For stability, the magnitude of the amplification factor must satisfy
Calculate
Using
To find the maximum
For stability, we require
Modified Equation Analysis#
While Von Neumann Stability Analysis provides insights into the stability of numerical schemes, Modified Equation Analysis delves deeper by examining the leading-order truncation errors introduced by discretization. This analysis reveals how numerical schemes can inadvertently introduce artificial diffusion or dispersion into the solution.
To determine how the Upwind Scheme modifies the original advection equation by introducing additional terms that represent numerical errors.
Express the Upwind Scheme Using Taylor Series Expansions
Start with the upwind update equation:
(539)#in addition to the grid point that we want to update,
, there are two additional grid points. They are and .Expand
and around the point using Taylor series:(540)#Using the shorthands
and , the above series can be written as(541)#
Substitute the Taylor Expansions into the Upwind Scheme
Substitute the expansions into the upwind update equation:
(542)#Because the derivatives are exact (instead of numerical), we can drop the functions’ evaluations at the dicrete points
and . Simplify the equation, we have:(543)#
Rearrange and Substitute the Original PDE
Taking the spatial and temporal derivatives of the original advection equation
, we have(544)#Combine, we obtain the two-way wave equation:
(545)#Substituting the wave equation into the modified equation, we obtain:
(546)#
Combine Like Terms
Put back the definition of
and rearrange,(547)#Define
be the numerical diffusion coefficient, the above equation reduces to(548)#
The Modified Equation Analysis reveals that the Upwind Scheme introduces an artificial diffusion term proportional to
However, this introduction of numerical diffusion comes with a trade-off in accuracy.
While the diffusion helps stabilize the solution, it also has the unintended effect of smearing out important features such as sharp interfaces or discontinuities within the advected quantity
Furthermore, the Modified Equation Analysis reinforces the critical importance of adhering to the Courant-Friedrichs-Lewy (CFL) condition
Modified Equation Analysis for the FTCS Scheme#
We performed the Von Neumann Stability Analysis last lecture, highlighting the instability of the FTCS scheme for the linear advection equation. Modified Equation Analysis provides further insights into the nature of the errors introduced by the numerical discretization, revealing how these errors affect the accuracy and stability of the numerical solution.
To determine how the Forward Time Centered Space (FTCS) scheme modifies the original linear advection equation by introducing additional terms that represent numerical errors, specifically focusing on artificial diffusion or dispersion.
Start with the FTCS Update Equation
Recalling the FTCS scheme for the linear advection equation is given by:
(549)#where:
is the numerical approximation of at spatial index and time level , is the constant advection speed, and are the time and spatial step sizes, respectively, is the Courant number.
Expand the Temporal and Spatial Terms Using Taylor Series
Expand
around :(550)#Expand
and around :(551)#
Substitute the Taylor Expansions into the FTCS Scheme
Substitute the expansions into the FTCS update equation:
(552)#Simplify,
(553)#
Substitute and Rearrange
Similar to the upwind screen, we recall that the advection equation implies the wave equation
. Taking an additional time derivative, we obtain:(554)#Substitute this into the modified equation, we obtain
(555)#Rearrange and drop the indices
and , the Final Modified Equation is:(556)#
The Modified Equation Analysis for the FTCS scheme has a second-order anti-diffusion term and a third-order dispersive term. These terms affect the numerical solution in several ways:
Numerical Instability: Unlike the Upwind Scheme, which introduces artificial diffusion to enhance stability, the FTCS scheme has an anti-diffusion term that is unstable.
Introduction of Dispersive Errors: The term
represents a dispersive error that causes different wave components to travel at slightly different speeds. This leads to phase errors where waveforms become distorted over time, deviating from the exact solution.Impact on Solution Accuracy: The introduced dispersive term does not counteract the amplification of numerical errors. Instead, it modifies the original equation in a way that can exacerbate inaccuracies, especially for higher-frequency components of the solution. Over time, these errors accumulate, leading to significant deviations from the true solution, as evidenced by the Von Neumann Stability Analysis.
Reinforcement of Von Neumann Stability Findings: The Modified Equation Analysis complements the Von Neumann Stability Analysis by providing a physical interpretation of why the FTCS scheme is unstable. The introduced dispersive errors contribute to the amplification of numerical oscillations, aligning with the conclusion that
for any .Practical Considerations: Practitioners must recognize that the FTCS scheme not only fails to maintain stability but also introduces errors that distort the solution without providing any compensatory benefits.
Consequently, the FTCS scheme is unsuitable for solving advection-dominated PDEs where both stability and accuracy in capturing wave propagation are essential.
Lax-Wendroff Scheme#
In numerical simulations of Partial Differential Equations (PDEs), achieving both accuracy and stability is essential. First-order schemes like the Upwind method are simple to implement but often introduce significant numerical diffusion, which can blur important features of the solution. To address these limitations, higher-order schemes have been developed to provide more accurate and reliable results.
The Lax-Wendroff Scheme is a second-order accurate finite difference method designed to solve hyperbolic PDEs, such as the linear advection equation. Unlike first-order methods, the Lax-Wendroff scheme incorporates both temporal and spatial derivatives up to the second order. This allows it to capture wave propagation more accurately while minimizing numerical diffusion and dispersion.
The main advantage of the Lax-Wendroff Scheme is its ability to maintain higher accuracy without compromising stability. By extending the Taylor series expansion to include second-order terms, the scheme reduces the smearing effect seen in first-order methods. Additionally, it better preserves the shape and speed of waves, making it suitable for problems where precise wave behavior is crucial.
However, the increased accuracy of the Lax-Wendroff Scheme comes with added complexity. The scheme requires careful implementation to ensure that the higher-order terms are correctly accounted for, and it may still exhibit oscillations near sharp gradients or discontinuities. Despite these challenges, the Lax-Wendroff Scheme remains a valuable tool in computational fluid dynamics and other fields requiring accurate wave propagation.
Derivation#
We begin by expanding
Substitute the advection equation and the wave equation into the Taylor series expansion:
and approximate the spatial derivatives using centered finite differences:
we obtain:
Simplify the equation to isolate
# Parameters
c = 1.0 # Advection speed
L = 1.0 # Domain length
T = 1.25 # Total time
nx = 100 # Number of spatial points
dx = L / nx # Spatial step size
dt = 0.005 # Time step size
nt = int(T / dt) # Number of time steps
# Courant number
sigma = c * dt / dx
print(f"Courant number (sigma): {sigma}")
# Stability condition (for Lax-Wendroff, no strict CFL condition, but accuracy improves with sigma <=1)
if sigma > 1:
warnings.warn(f"Courant number sigma = {sigma} > 1. Accuracy may decrease.")
# Spatial grid
x = np.linspace(0, L, nx, endpoint=False)
u_initial = np.sin(2 * np.pi * x) # Initial condition: sinusoidal wave
# Initialize solution array
u = u_initial.copy()
# Time-stepping loop using Lax-Wendroff scheme
for n in range(nt):
u_new = np.zeros_like(u)
for i in range(nx):
u_new[i] = (u[i]
- 0.5 * sigma * (u[(i+1)%nx] - u[(i-1)%nx])
+ 0.5 * sigma**2 * (u[(i+1)%nx] - 2*u[i] + u[(i-1)%nx]))
u = u_new
# Analytical solution
u_exact = np.sin(2 * np.pi * (x - c * T))
# Plotting the results
plt.figure(figsize=(12, 6))
plt.plot(x, u_initial, label='Initial Condition', linestyle='--')
plt.plot(x, u_exact, label='Exact Solution', linewidth=2)
plt.plot(x, u, label='Lax-Wendroff Scheme', linestyle=':', linewidth=2)
plt.xlabel('x')
plt.ylabel('u')
plt.legend()
plt.grid(True)
Courant number (sigma): 0.5
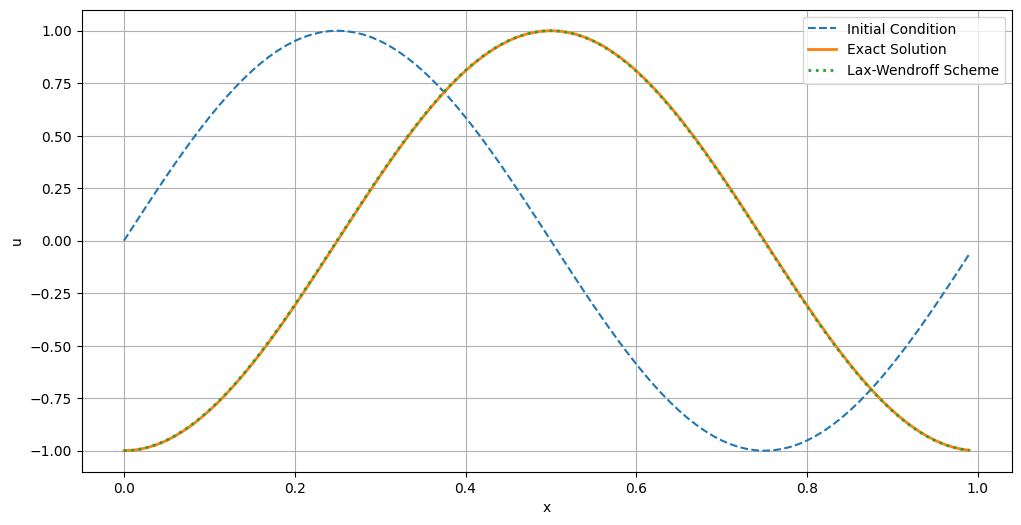
Non-Dimensionalization and Key Dimensionless Numbers#
Non-dimensionalization is a fundamental technique in the analysis of partial differential equations (PDEs) and fluid dynamics. By converting variables and equations into dimensionless forms, researchers and engineers can simplify complex systems, identify dominant physical effects, and generalize solutions across different scales. This section explores the purpose of non-dimensionalization, introduces key dimensionless numbers—Reynolds Number (Re), Mach Number (Ma), and Prandtl Number (Pr)—and discusses their applications and significance in various physical systems.
Purpose of Non-Dimensionalization#
Non-dimensionalization serves several critical purposes in the analysis and solution of PDEs:
Simplifying Equations for Analysis
By scaling variables and parameters, non-dimensionalization reduces the number of independent variables and parameters in the governing equations. This simplification often transforms complex, dimensional equations into a more manageable, dimensionless form. For instance, consider the non-dimensionalization of the Navier-Stokes equations:
(562)#Here, the introduction of dimensionless variables
, , and has reduced the complexity of the original equations by consolidating physical parameters into dimensionless groups like the Reynolds Number (Re).Identifying Dominant Physical Effects in Specific Regimes
Non-dimensionalization reveals the relative importance of various physical phenomena within a system. By examining the dimensionless numbers that emerge from the process, one can determine which terms in the equations are significant and which can be neglected under certain conditions. This identification is crucial for developing approximate solutions and understanding the behavior of the system in different regimes.
For example, in high Reynolds number flows (Re
), inertial forces dominate over viscous forces, simplifying the Navier-Stokes equations by reducing the influence of the viscous term. Conversely, in low Reynolds number flows (Re ), viscous forces are predominant, and inertial terms can often be neglected.
Key Dimensionless Numbers#
Several dimensionless numbers play pivotal roles in fluid dynamics and the study of PDEs. Among the most important are the Reynolds Number (Re), Mach Number (Ma), and Prandtl Number (Pr). Each of these numbers encapsulates the ratio of different physical effects, providing insight into the system’s behavior.
Reynolds Number (Re): Ratio of Inertial to Viscous Forces#
The Reynolds Number is defined as:
where:
is the fluid density, is a characteristic velocity, is a characteristic length scale, is the dynamic viscosity, is the kinematic viscosity.
Physical Interpretation:
Reynolds Number quantifies the relative significance of inertial forces (associated with the fluid’s motion) to viscous forces (associated with the fluid’s internal friction). A high Re indicates that inertial forces dominate, leading to turbulent flow, while a low Re suggests that viscous forces are more influential, resulting in laminar flow.
Applications:
Flow Regimes: Determining whether a flow will be laminar or turbulent based on Re.
Scale Modeling: Ensuring dynamic similarity in wind tunnel experiments by matching Re between models and real-world scenarios.
Astrophysical Flows: In stellar interiors, high Re can lead to turbulent convection, influencing energy transport and stellar evolution.
Mach Number (Ma): Ratio of Flow Velocity to Speed of Sound#
The Mach Number is defined as:
where:
is the characteristic flow velocity, is the speed of sound in the fluid.
Physical Interpretation:
Mach Number measures the compressibility effects in a flow. When Ma
Applications:
Aerodynamics: Designing aircraft and rockets by analyzing flow behavior at different Mach regimes.
Astrophysics: Studying phenomena like shock waves in supernova explosions and supersonic jets from active galactic nuclei.
Explosive Events: Understanding the propagation of shock waves generated by explosions.
Prandtl Number (Pr): Ratio of Momentum Diffusivity to Thermal Diffusivity#
The Prandtl Number is defined as:
where:
is the kinematic viscosity, is the thermal diffusivity, is the thermal conductivity, is the specific heat at constant pressure.
Physical Interpretation:
Prandtl Number compares the rate at which momentum diffuses through a fluid to the rate at which heat diffuses. A low Pr indicates that heat diffuses rapidly compared to momentum, while a high Pr suggests that momentum diffuses more quickly than heat.
Applications:
Heat Transfer: Designing heat exchangers and cooling systems by understanding the relative rates of heat and momentum transfer.
Boundary Layer Analysis: Determining the thickness of thermal and velocity boundary layers in fluid flows.
Astrophysical Processes: Modeling heat conduction in stellar atmospheres where Pr influences energy transport mechanisms.
Applications of Dimensionless Numbers#
Dimensionless numbers like Re, Ma, and Pr are instrumental in analyzing and predicting the behavior of physical systems across various domains:
Flow Control and Design
In engineering, these numbers guide the design of systems involving fluid flow. For example, ensuring that aircraft operate efficiently at desired Reynolds and Mach numbers is crucial for performance and safety.
Scale Modeling and Similitude
In experimental studies, ensuring that the dimensionless numbers of a model match those of the real system (dynamic similarity) allows for accurate predictions and validations of theoretical models through physical experiments.
Astrophysical Fluid Systems
In astrophysics, dimensionless numbers help in scaling and understanding fluid behaviors in vastly different environments:
Stellar Convection: High Reynolds numbers indicate turbulent convection currents within stars, affecting energy transport and mixing of stellar material.
Accretion Disks: Mach numbers determine the compressibility of gas in accretion disks around black holes, influencing the formation of shock waves and jet structures.
Interstellar Medium: Prandtl numbers aid in modeling the thermal and momentum diffusion in the diffuse interstellar gas, impacting star formation rates and the dynamics of molecular clouds.
Historical Anecdote: Fermi’s Estimation of Atomic Bomb Energy Release#
One of the most illustrative examples of the power of non-dimensionalization and dimensional analysis in physics is Enrico Fermi’s remarkable estimation of the energy released during the first atomic bomb test, known as the Trinity test, conducted on July 16, 1945. This anecdote not only highlights Fermi’s ingenuity but also demostrate the practical utility of dimensionless numbers and scaling laws in tackling complex, real-world problems with limited empirical data.
During World War II, the Manhattan Project was a top-secret initiative aimed at developing nuclear weapons. As the project progressed, scientists faced the daunting challenge of predicting the yield of the atomic bombs they were designing. Precise calculations were hindered by the lack of comprehensive empirical data, necessitating innovative approaches to estimate explosive power accurately.
Enrico Fermi, a renowned physicist known for his ability to make quick, accurate estimates with minimal data, was tasked with predicting the energy release of the impending Trinity test. His method exemplified dimensional analysis—a technique that uses the fundamental dimensions (such as mass, length, and time) to derive relationships between physical quantities.
Fermi’s estimation process involved the following steps:
Observing the Shock Wave: After the detonation, Fermi and his colleagues observed the speed at which the shock wave propagated through the air. Let’s denote this speed as
.Estimating the Shock Wave Radius: By timing how long it took for the shock wave to reach a certain distance from the explosion site, Fermi estimated the radius
of the blast wave after a specific duration .Applying the Sedov-Taylor Scaling: The Sedov-Taylor solution describes the propagation of a strong shock wave from a point explosion in a homogeneous medium. According to this theory, the energy
of the explosion is related to the shock wave’s speed , radius , and the density of the surrounding air by the following relationship:(566)#This equation is derived by balancing the kinetic energy imparted to the air by the shock wave with the energy of the explosion.
Calculating the Energy: By substituting the estimated values of
, , and into the above equation, Fermi arrived at an approximate value for the energy released during the explosion.
There is an arXiv paper on this topic.